Expressions to assign values to variables
Number=0 |
Expressions to return values using arithmetic operations (+ – * / ^ mod)
Average=total/5 |
Execution of lines of code in sequence demonstrating input – process- output
1. SEND “Enter First Number” TO DISPLAY 2. RECEIVE first_number FROM (INTEGER) KEYBOARD [input] 3. SEND “Enter Second Number” TO DISPLAY 4. RECEIVE second_number FROM (INTEGER) KEYBOARD [input] 5. SET total TO first_number + second_number [process] 6. SEND total TO DISPLAY [output] |
Expressions to concatenate strings and arrays using the & operator
Bob Smith is an example of concatenation
firstName=”Bob” secondName=”Smith” print(firstName + secondName)
BobSmith |
Use of selection constructs including simple and complex conditional statements and logical operators.
This is a simple statement as there is one conditions 1. IF pupil_mark >=50 THEN 2. SEND pass message TO DISPLAY 3. ELSE 4. SEND fail message TO DISPLAY 5. END IF
This is a complex statement as there are two conditions 1. IF pupil_mark >=50 AND assessments_mark = 100 THEN 2. SEND pass message TO DISPLAY 3. ELSE 4. SEND fail message TO DISPLAY 5. END IF |
Iteration and repetition using fixed and conditional loops
Fixed loop below as it will loop a fixed number of times depending on the users answer number=int(input(“How many smart phones?”)) total=0.0 for phone in range(number): Name=input(“Please enter the name of the smart phone:”) cost=float(input(“Please enter the cost of the smart phone:”)) total=total + cost print(“Total cost = “,total)
Below is a Conditional Loop as it depends whether the user has any money left. money=int(input(“Please enter your pocket money in £”)) while money>0: name=input(“Please enter the name of the CD you want to buy”) cost=float(input(“Please enter the cost of the CD”)) money=money-cost print(“You have ran out of pocket money”) |
Pre-defined functions (with parameters) – round(22.34 ) will round numbers 22.34 becomes 22 or sqrt(4) will get the square root 2, or 9 will become 3. |
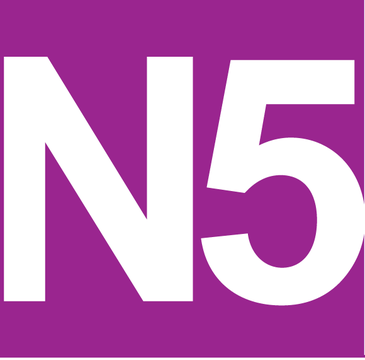