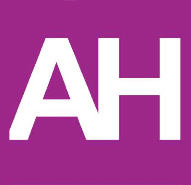
Reading Form Data
Data from HTMl forms can be sent using GET or POST methods.
PHP needs to use the correct commands to match the method used.
1 2 | $forename = $_POST [ "firstname" ]; $surname = $_POST [ "lastname" ]; |
1 2 | $forename = $_GET [ "firstname" ]; $surname = $_GET [ "lastname" ]; |
Either method can be used by checking the method used:
1 2 3 4 5 6 7 | if ( $_SERVER [ "REQUEST_METHOD" ] == "POST" ) { $forename = $_POST [ "firstname" ]; $surname = $_POST [ "lastname" ]; } elseif ( $_SERVER [ "REQUEST_METHOD" ] == "GET" ) { $forename = $_GET [ "firstname" ]; $surname = $_GET [ "lastname" ]; } |
Checking for Existence of values
HTML forms only send data that has been entered, or given default values.
A form may have optional data input items, and trying to read these will cause the PHP program to stop. If this is a possibility, then check that the values exist first:
1 2 3 4 | forename = "" ; if (isset( $_POST [ "firstname" ])) { $forename = cleanInput( $_POST [ "firstname" ]); } |
This can be written, at the expense of readability as:
1 | $forename = isset( $_GET [ 'firstname' ]) ? $_GET [ 'firstname' ] : '' ; |
Pre-Processing Form Data
Form data will often used to create SQL queries, with the potential for SQL injection attacks.
Although not a requirement for the AH course, clearing form data of any unwanted characters may be useful for project work.
1 2 3 4 5 6 7 8 9 | function cleanInput( $data ) { $data = trim( $data ); $data = stripslashes ( $data ); $data = htmlspecialcharacters( $data ); return $data } $forename = cleanInput( $_POST [ "firstname" ]); $surname = cleanInput( $_POST [ "lastname" ]); |