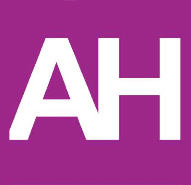
Reading Form Data
Data from HTMl forms can be sent using GET or POST methods.
PHP needs to use the correct commands to match the method used.
$forename = $_POST["firstname"]; $surname = $_POST["lastname"];
$forename = $_GET["firstname"]; $surname = $_GET["lastname"];
Either method can be used by checking the method used:
if ($_SERVER["REQUEST_METHOD"] == "POST") { $forename = $_POST["firstname"]; $surname = $_POST["lastname"]; } elseif ($_SERVER["REQUEST_METHOD"] == "GET") { $forename = $_GET["firstname"]; $surname = $_GET["lastname"]; }
Checking for Existence of values
HTML forms only send data that has been entered, or given default values.
A form may have optional data input items, and trying to read these will cause the PHP program to stop. If this is a possibility, then check that the values exist first:
forename = ""; if (isset($_POST["firstname"])) { $forename = cleanInput($_POST["firstname"]); }
This can be written, at the expense of readability as:
$forename = isset($_GET['firstname']) ? $_GET['firstname'] : '';
Pre-Processing Form Data
Form data will often used to create SQL queries, with the potential for SQL injection attacks.
Although not a requirement for the AH course, clearing form data of any unwanted characters may be useful for project work.
function cleanInput($data) { $data= trim($data); $data = stripslashes($data); $data = htmlspecialcharacters($data); return $data } $forename = cleanInput($_POST["firstname"]); $surname = cleanInput($_POST["lastname"]);