This program is designed to allow the Computer to get to know the user. This is often seen at the start of a game where the user would enter a name for their character. This will then be used throughout the game to add a personal touch.
You can see the code and output below, or you can access it on repl.it by clicking here.
Code
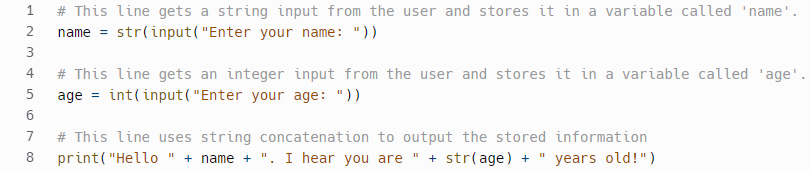
Output

New Constructs/Concepts
Variables
In programming, we often need to store data in memory. In order to do this, we use a ‘variable’. Recall from the Computer Systems unit that memory is organised into individual locations with a unique memory address. A variable allows us to allocate a chunk of this memory and give it a name. The computer then knows that any time we use that name, we are referring to the data that’s stored in the allocated chunk of memory. The data that we store in that chunk of memory can change throughout the program.
Variables have a name, a type and a value.
The name is the identifier we give the variable in order to refer to it throughout our program. In Program 2, we call our variables ‘name’ and ‘age’. This is sensible as it’s clear to the developer and anybody else that reads the code what information is being stored in that variable.
The type is the kind of data that the variable is storing. The following types are common across programming languages:
- Character – A single letter, number or symbol – eg: S, 4, £, +
- String – A collection of characters (words, sentences) – eg: “Hello World”
- Integer – A whole number – eg: 4, 6, 10
- Real – A number with a fractional part (a decimal) – eg: 4.5, 3.14, 5.4394
- Boolean – A value which is either true/false or on/off
In Python, there is no such thing as a ‘character’ data type, we simply use string.
Some programming languages require variables to be declared (given a name and a type) before they are given a value. However, in Python, we can create a variable on the same line of code that we give it a value. Giving a variable a value is called variable assignment – this is shown on lines 2 and 5 of this program.
Construct | Line(s) | Python Syntax | Purpose |
---|---|---|---|
Variable Assignment | 2, 5 | = | To store a value in a variable (chunk of memory with a given name) |
Input
Programs require input all the time, using input devices such as the mouse or keyboard. In our first basic programs, we will learn how to handle input from the keyboard as shown in this program – for example on lines 2 and 5 of this program, the user is asked to enter their name and age.
In some programming languages, we do not have to take any regard to the type of information that is being inputted as the variable it is being stored in will have been given a type when it was declared. However as Python is dynamically typed (variable types can change throughout the program), we need to state the type every time we get input. We can see this in this program:
When we are getting the name, str(input()) is used – str meaning string (text). When we are getting the age, int(input()) is used – int being short for integer (whole number). We can also use bool(input()) for boolean values. For real numbers, we use float(input()) – this is because ‘floating point representation’ is used to represent decimal numbers in a computer.
The phrase that we put inside the brackets after ‘input’ is what the user will be shown before they enter their input.
String Concatenation
We will often have to join strings together to create a longer and more meaningful output. To do this we use ‘string concatenation’. In Python, this involves using the ‘+’ symbol. This can be seen on line 8 of this program.
Construct | Line(s) | Python Syntax | Purpose |
---|---|---|---|
String Concatenation | 8 | + | To join strings together |
Parsons Problem
Drag and drop the following lines of code to create a program which asks the user for their name, age and shoe size. It should then display their name and age followed by the size of shoes they should be looking for.