Dry run
A dry run is the process of a programmer manually working through their code to trace the value of variables. Usually, a dry run would involve a printout of the code and the developer working through it line by line to check the value of a variable at each stage.
Dry runs are useful in detecting logic errors where the code executes without throwing any exceptions but the result is not the expected value.
Trace tables are used to allow programmers to trace the value of variables as each line of code is executed. The values of the variables are displayed in a table and assist the programmer in identifying any potential errors.
Example
This algorithm is designed to count the number of potential astronauts who qualify for training based upon the height requirements of a space agency. Astronauts need to be between 1.57 and 1.91 metres tall inclusive of both heights.
total = 0
all_heights = [1.87, 1.48, 1.57, 1.91, 2.01]
for height in all_heights:
if height > 1.57 and height <= 1.91:
total += 1
There is a deliberate error on line 4 of this reference language example. The symbol > has been used instead of >= before the number 1.57.
This will allow us to look at a trace table to identify the point at which the program failed to return an expected value. Three values from the all_heights array should be accepted as they fall within the desired range. The values that should trigger an increment to the total are 1.87, 1.57 and 1.91.
You will notice that after the first iteration of the loop the program will return to line 3. Lines 3 to 5 are repeated for each height in the 1-D array all_heights. The trace table shows a complete run through of the values stored by each variable during the execution of the algorithm.
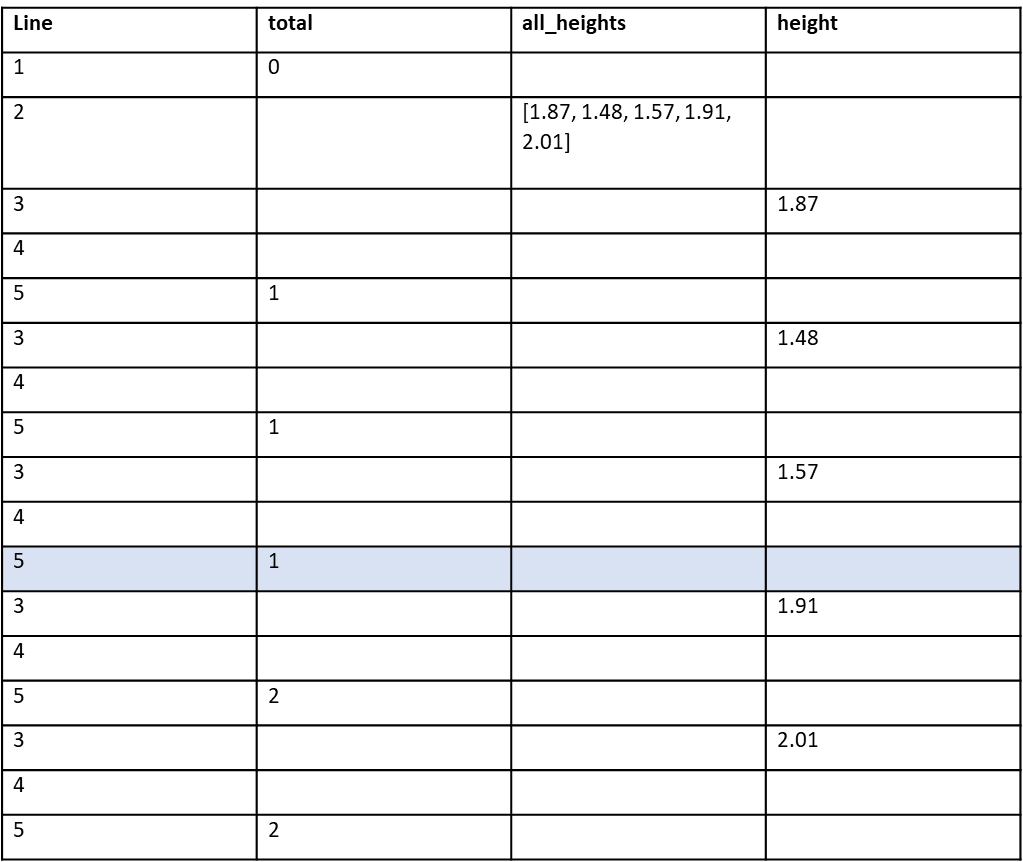
On the third iteration of the loop a height of 1.57 should have incremented the total variable by 1. Changing it from 1 to 2. The trace table highlights that this did not occur so we have found the logic error. To fix the issue we change the > to be >=.
Breakpoints
When a programmer creates code, they can add what is known as a breakpoint. A breakpoint is a point in the program where the code will stop executing.
For example, if the programmer amended the logic error in the trace table example, they may wish to trigger a break point at line 5 in the algorithm.
The breakpoint would force the algorithm to stop running each time the loop reaches line 5.
When a breakpoint is set and triggered the program will display the value of the variables at that point only. This would allow the programmer to check that the value.
In large programs it is not uncommon to use a trace table prior to setting breakpoints. A trace table will highlight the fact that the value is not as it should be, and the programmer can then set breakpoints to check specific parts of the program without having to trace each variable all of the time.
In Python IDLE you can set a breakpoint by right clicking on the line of code and choosing the breakpoint option. Then choosing the Step option in the Debug window to go through the code line by line. The Debug window also allows you to choose whether to watch local or global variables.
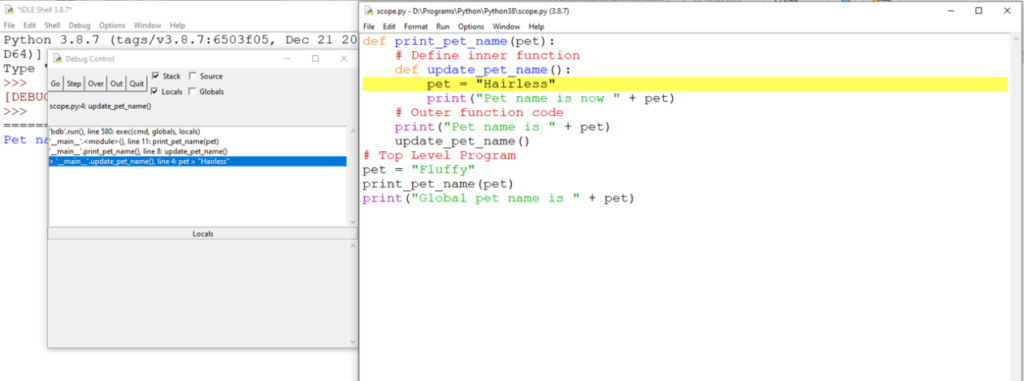
In Repl.it in the left hand sidebar under Tools there is a Debugger option where you can use breakpoints and step through the code.
Watchpoints
Where a breakpoint is triggered at a specific point in the execution of code, a watchpoint is concerned with changes in the value of specific variables. For example, you could create a watchpoint on a variable called score and apply a condition to the watchpoint that stops execution when the value of score changes to ten. This would allow you to check that the value changes at the point in the execution that you expect it to.
You can also use watchpoints in conjunction with breakpoints to look at the value of only specific variables when a breakpoint is triggered.
Watchpoints are not a built in option in Python IDLE but you can mimic the result by placing a print() line to show the value of the variable. Once error has been located and fixed you can remove these temporary print() lines.