A while loop continuously loops until a conditional check is no longer True.
The code:
counter = 0
while counter < 5:
print(counter)
counter += 1
Gives an output:
0
1
2
3
4
In this example the loop checks if the counter variable is less than 5. So long as that check returns a boolean True then the code inside the loop will run.
Be careful with while loops to avoid infinite looping. In the code below the programmer forgot the line that changes the value of counter. As such it always stays at 0 which will never be equal or greater than 5.
counter = 0
while counter < 5:
print(counter)
Break
Just as before with the for loop, the break keyword will stop the loop and go to the first line of code after it.
The code:
counter = 0
value_to_check = 3
while counter < 5:
print(counter)
if value_to_check == counter:
break
counter += 1
Gives an output:
0
1
2
3
Continue
Same as with the for loop but remember when the continue line is encountered, the program jumps back to the start of the loop section. Because of this it is important to remember to change the value used in the conditional check before the continue line if using a While loop. Otherwise the program can get stuck in an infinite loop.
Good
counter = 0
value_to_check = 3
while counter < 5:
counter += 1
if value_to_check == counter:
continue
print(counter)
Bad
counter = 0
value_to_check = 3
while counter < 5:
if value_to_check == counter:
continue
counter += 1
print(counter)
In the bad example, when the counter is equal to 3 the continue line runs. So it skips to the start of the loop, but the counter is still 3. It’s stuck in an infinite loop.
Design
So how do represent the above code. Pretty much the same as a for loop. Just change the description in the decision box. But notice the lines where we add a number to our counter. That is neither a decision, initialisation or an input/output. We need a new shape for the flowchart.
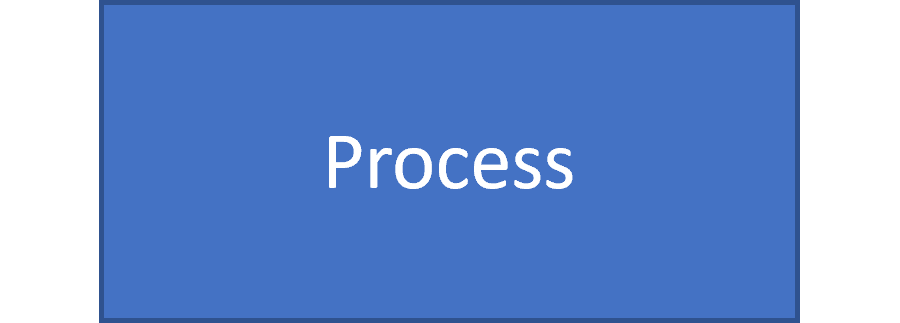
The process box can be used to represent one or more lines covering anything from simple arithmetic to function calls. If another shape doesn’t cover what you need to do, then use a process box.
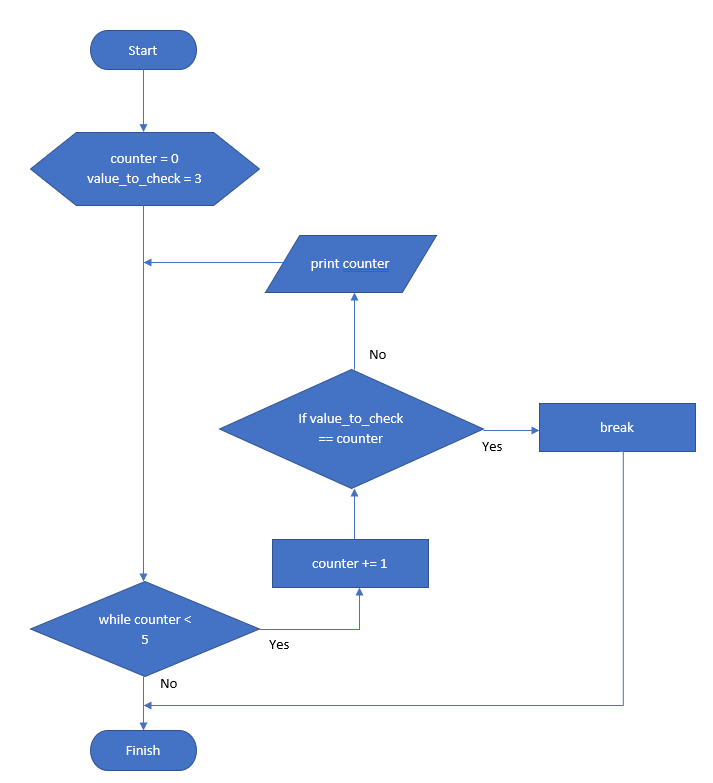