The <a> tag defines a hyperlink, which is used to link from one page to another. It isn’t just used for text, you can put an anchor around anything, but I’m introducing it here so we can use it in the menu.
The most important attribute of the <a> element is the href attribute, which indicates the link’s destination.
By default, links will appear in different colours depending on if the page has been visited or is active.
If the <a> tag has no href attribute, it is only a placeholder for a hyperlink.
A linked page is normally displayed in the current browser window, unless you specify another target.
<!DOCTYPE html>
<html lang="en">
<head>
<link rel="stylesheet" href="/style.css">
<script type="text/javascript" src="/script.js"></script>
<meta charset="UTF-8">
<meta author="Your name here">
<title>My first webpage</title>
</head>
<body>
<header>
This is my website name
</header>
<nav>
<a href="/index.html">Home</a>
</nav>
<main>
This is the main part of the page.
</main>
<footer>
This is the footer.
</footer>
</body>
</html>
The <h1> to <h6> tags are used to define HTML headings.
<h1> defines the most important heading. <h6> defines the least important heading.
Note: Only use one <h1> per page – this should represent the main heading/subject for the whole page. Also, do not skip heading levels – start with <h1>, then use <h2>, and so on.
<!DOCTYPE html>
<html lang="en">
<head>
<link rel="stylesheet" href="/style.css">
<script type="text/javascript" src="/script.js"></script>
<meta charset="UTF-8">
<meta author="Your name here">
<title>My first webpage</title>
</head>
<body>
<header>
This is my website name
</header>
<nav>
<a href="/index.html">Home</a>
</nav>
<main>
<h1>Welcome to my home page</h1>
</main>
<footer>
This is the footer.
</footer>
</body>
</html>
The <p> tag defines a paragraph.
Because <p> tags are block elements, browsers automatically add a single blank line before and after each <p> element.
<!DOCTYPE html>
<html lang="en">
<head>
<link rel="stylesheet" href="/style.css">
<script type="text/javascript" src="/script.js"></script>
<meta charset="UTF-8">
<meta author="Your name here">
<title>My first webpage</title>
</head>
<body>
<header>
This is my website name
</header>
<nav>
<a href="/index.html">Home</a>
</nav>
<main>
<h1>Welcome to my home page</h1>
<p>This is the main part of the page.</p>
</main>
<footer>
This is the footer.
</footer>
</body>
</html>
The <br> tag inserts a single line break.
The <br> tag is useful for writing addresses or poems.
The <br> tag is an empty tag which means that it has no end tag.
<!DOCTYPE html>
<html lang="en">
<head>
<link rel="stylesheet" href="/style.css">
<script type="text/javascript" src="/script.js"></script>
<meta charset="UTF-8">
<meta author="Your name here">
<title>My first webpage</title>
</head>
<body>
<header>
This is my website name
</header>
<nav>
<a href="/index.html">Home</a>
</nav>
<main>
<h1>Welcome to my home page</h1>
<p>This is the main part of the page.</p>
<h2>To do list</h2>
<p>
Learn HTML<br>
Learn CSS<br>
Learn Javascript<br>
Make a website
</p>
</main>
<footer>
This is the footer.
</footer>
</body>
</html>
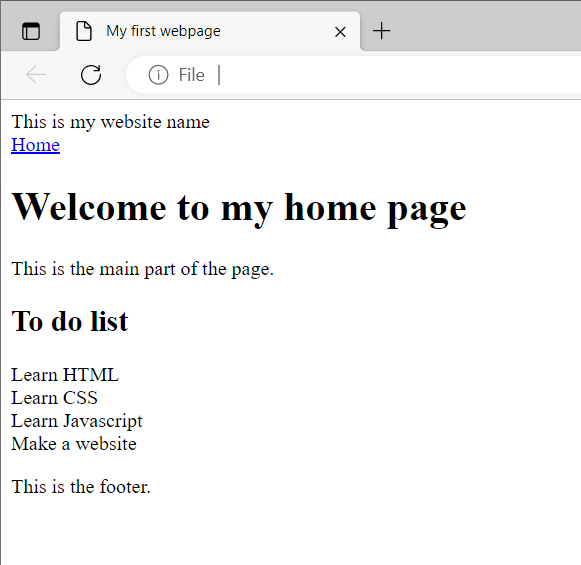
Hang on, that’s a list. There are better ways to do a list than using line breaks. We can use the <ul>, <ol>, and <li> tags.
They can contain more than simply text. Very useful when grouping similar content such as in a menu or gallery.
The <ol> tag defines an ordered list. An ordered list can be numerical or alphabetical.
- This
- is
- an
- ordered
- list
The <ul> tag defines an unordered (bulleted) list.
- This
- is
- an
- unordered
- list
The <li> tag defines a list item.
In <ul> the list items will usually be displayed with bullet points.
In <ol> the list items will usually be displayed with numbers or letters.
<!DOCTYPE html>
<html lang="en">
<head>
<link rel="stylesheet" href="/style.css">
<script type="text/javascript" src="/script.js"></script>
<meta charset="UTF-8">
<meta author="Your name here">
<title>My first webpage</title>
</head>
<body>
<header>
This is my website name
</header>
<nav>
<a href="/index.html">Home</a>
</nav>
<main>
<h1>Welcome to my home page</h1>
<p>This is the main part of the page.</p>
<h2>To do list</h2>
<ul>
<li>Learn HTML</li>
<li>Learn CSS</li>
<li>Learn Javascript</li>
<li>Make a website</li>
</ul>
</main>
<footer>
This is the footer.
</footer>
</body>
</html>
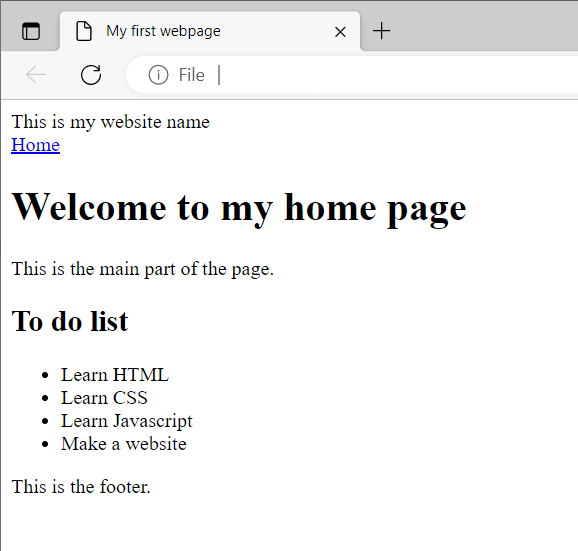
Much better.
Style vs substance
If you’ve ever looked at HTML code before, you might have seen bold <b> or italic <i> tags.
Of maybe you’ve seen strong <strong> or emphasis <em> tags.
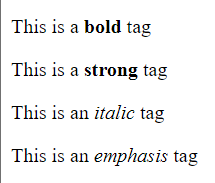
What’s the difference? They look the same. Bold and italic are just about making the text look different. Strong and emphasis place importance and emphasis on the text wrapped in the tags. Someone using a screen reader or a text to speech system because of visual impairments will have strong and emphasised words read out differently.
It is best to either forget about <b> and <i> tags, or think of them as a short hand notation for styling text. You could get the same effect using the <span> tag with some CSS.
The <span> tag is an inline container used to mark up a part of a text, or a part of a document. They can be easily styled by CSS or manipulated with JavaScript using the class or id attribute.
The <span> tag is much like the <div> element, but <div> is a block-level element and <span> is an inline element.
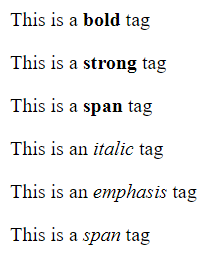
<p>This is a <b>bold</b> tag</p>
<p>This is a <strong>strong</strong> tag</p>
<p>This is a <span style="font-weight:bold">span</span> tag</p>
<p>This is an <i>italic</i> tag</p>
<p>This is an <em>emphasis</em> tag</p>
<p>This is a <span style="font-style:italic">span</span> tag</p>
Basically, use HTML to categorise the content of a website. Use CSS to style the content. Your HTML is just saying “This is a menu”, “This is a block of text” and so on.