A common requirement in programming is to be able to repeat an action a given number of times, or once for each entry in a list.
A For loop allows you to do this. The name basically means “For each entry, do this code”.
A for loop goes element by element through a list. The current element of the list is stored in a variable defined in the for loop. The format is:
for x in y:
Where x is the new variable that will hold the contents of the current list element. And y is the list that you are looping through.
The code:
print("Program starts")
list_of_animals = [ "cat", "dog", "rabbit"]
for animal in list_of_animals:
print(animal)
print("Program ends")
Gives an output:
Program starts
cat
dog
rabbit
Program ends
In each time through the loop the variable animal is set to the next element in the list_of_animals array. So on the first iteration the value of animal is “cat”, the second time it is overwritten with “dog” and so on.
Just as you saw with the conditional statements the for line ends in a colon and all the code to be ran each time through the loop is indented.
Range
The range function generates a list of integers. This is particularly useful in for loops if you want to carry out a block of code a set number of times.
The code:
for counter in range(5):
print(counter)
Gives an output:
0
1
2
3
4
All that range does it return a list of numbers that start at 0 and counter up to (but never reach) the number provided to the function in the brackets.
So far we have looped through a list (technically range is its own iterator of type range), but a dictionary behaves differently because it is made up of key:value pairs.
If you just did:
exam_grades = {'JDoe': 85, 'MSmith': 67, 'BMacleod': 81}
for entry in exam_grades:
print(entry)
You would get:
JDoe
MSmith
BMacleod
Only the keys were printed.
To get the values you need to use:
exam_grades = {'JDoe': 85, 'MSmith': 67, 'BMacleod': 81}
for key,value in exam_grades.items():
print(key, value)
You would get:
JDoe 85
MSmith 67
BMacleod 81
Break
A break statement allows you to stop the current loop before the natural end. When the break line is encountered, the program continues from the first line after the end of the loop.
The code:
value_to_check = 3
for counter in range(5):
print(counter)
if value_to_check == counter:
break
Gives an output:
0
1
2
3
Here we can see the counter counting up from 0. Each time through the loop it will have 1 added to it. When it equals 3 though the if statement returns True and a break line is run. This stops the loop before the natural end.
Continue
A Continue Statement allows you to skip the remaining lines of code in the current time through a loop section. When the continue line is encountered, the program jumps back to the start of the loop section.
The code:
value_to_check = 3
for counter in range(5):
if value_to_check == counter:
continue
print(counter)
Gives an output:
0
1
2
4
You can see that 3 has been skipped. When the if statement condition returned True the continue line was run, this then exited that time around the loop which skipped the print(counter) line.
Design
So how do we represent a for loop in a flow chart? There are multiple ways to do it. You could go into a lot of detail and show the initial value of the loop, how it changes each time through and what the decision is going to be checking. Or simply put the for loop description into the decision box directly. It will be clear enough to yourself making the program or any tutors how many times that loop will run.
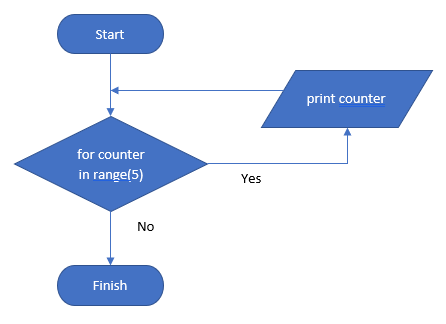
Now your flow chart has a visual loop so that it is clear there is a looped bit of code in your program. Any lines of code in your for loop happen between the decision and where it joins back to the main flow just before the decision.
What about when we are using an existing list rather than one made by range? Time for a new flowchart shape.
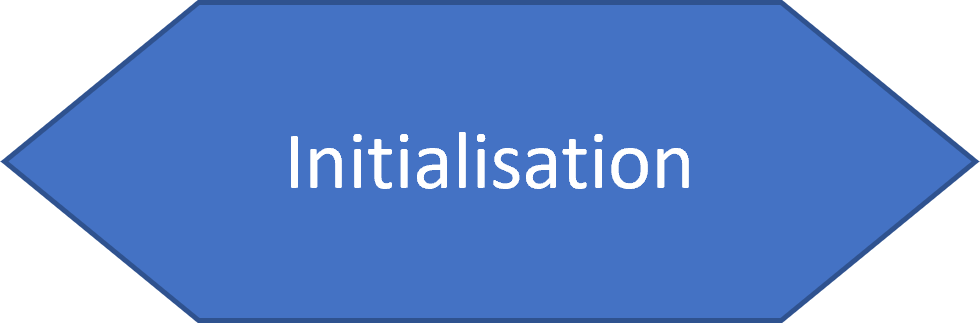
You can use this to initialise or declare variables or other data structures.
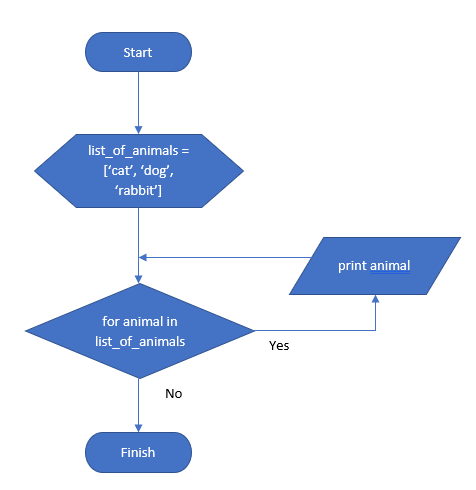
Just make sure the loop line goes back to just before the decision so that you aren’t re-initialising the variable.