Javascript is a form of client-side scripting, meaning scripting that runs on the client rather than the server. The client is the computer system (including tablets and mobile devices) which is running the web browser.
Client-side scripting can be used to make web pages change after they arrive at the browser.
Client side scripts can also be used to perform validation of data entered into forms.
Client-side scripts are:
- interpreted by the browser
- executed on the client system
JavaScript is the main client-side scripting language that can be used to create, delete, and manipulate HTML elements. You can type the Javascript code between script tags.
<script>
Code goes here
</script>
Extensive JavaScript code is often contained within its own file and linked to a webpage. This way the same code can be called from multiple webpages.
<script src="script.js"></script>
Some common tasks that can be performed using JavaScript relate to how the webpage responds to action taken by the user using their mouse or touchscreen.
These are called mouse events.
Using JavaScript, functions are created that will change what appears on screen depending upon what the user does with the mouse.
There are a number of mouse events that are used in HTML code to trigger the execution of JavaScript functions.
- onmouseout
- onmouseover
- onmousedown
- onmouseup
- onclick
- onmousemove
onmouseout
The onmouseout event occurs when the cursor is moved away from an element such as a button or a heading.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Javascript test</title>
<style>
#demo {
height: 10em;
width: 10em;
border: 1px solid black;
}
</style>
<script type="text/javascript">
function mouseOut() {
document.getElementById("demo").style.backgroundColor = "red";
}
</script>
</head>
<body>
<header><h1>Javascript test<h1></header>
<main>
<div id="demo" onmouseout="mouseOut()"> </div>
</main>
</body>
</html>
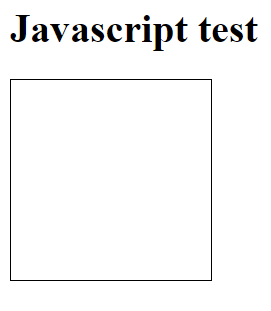
Move your mouse into the square and then out of it.
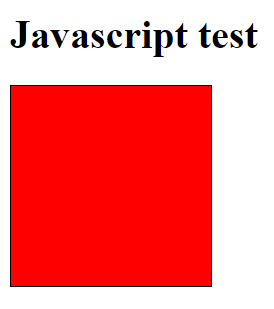
The background colour of the square should change to red.
Here there is an event listener attached to the demo div.
onmouseout="mouseOut()"
onmouseout is the name of the listener, it triggers an event On the action of the Mouse going Out of the element. The value in the quotes is what happens on this event. In this code it calls the function called mouseOut, just like in Python you can tell it is a function call by the brackets. The brackets are empty so we aren’t passing any values into it.
In the Javascript mouseOut function we only have one line:
document.getElementById("demo").style.backgroundColor = "red";
This is setting a new value to an entry in the Document Object Model (DOM) of the page. When a web page is loaded, the browser creates a Document Object Model of the page. This tree like structure contains all the elements of the page nested within each other, these elements might have attributes like href, height, id, class and so on, and values like the text contained in a link or paragraph.
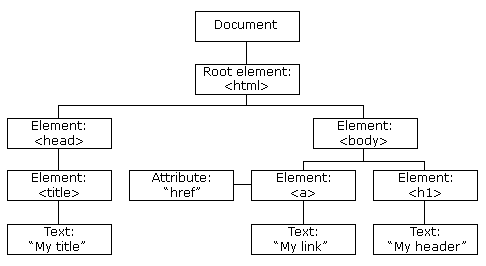
Using the getElementById Javascript function you can access the matching element in the DOM structure.
document.getElementById("demo")
This accesses the object that contains all the HTML details for the element that matches the id provided. In this case demo.
document.getElementById("demo").style.backgroundColor
Using these objects, you can access attributes assigned to them such as style. Then within that view and alter the backgroundColor property of it. Notice the naming is different from CSS; backgroundColor versus background-color.
If the object is an image, you can alter the image used with the src property. For example, to change the source image to test-image-large.png you could use:
document.getElementById("image1").src = "images/test-image-large.png"
onmouseover
The onmouseover event occurs when the cursor is hovered over an element such as a button or a heading. Add the following Javascript to either your HTML file or separate script file.
function mouseOver() {
document.getElementById("demo").style.backgroundColor = "blue";
}
Then update your demo div to include this new event:
<div id="demo" onmouseout="mouseOut()" onmouseover="mouseOver()"> </div>
Reload your page. Now when you mouse goes over the square the background colour should be set to blue, it will still turn red when you move out of the square.
onmousedown
The onmousedown event occurs when the user presses a mouse button over an element. Add the following Javascript to either your HTML file or separate script file.
function mouseDown() {
document.getElementsByTagName("main")[0].style.backgroundColor = "#123456";
}
Then update your demo div to include this new event:
<div id="demo" onmouseout="mouseOut()" onmouseover="mouseOver()" onmousedown="mouseDown()"> </div>
Save your code and reload your page and then click inside the square. The background colour of the main element should change to a dark blue.
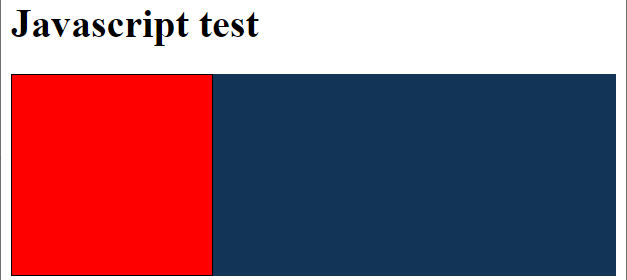
The getElementsByTagName function allows you to find all the DOM elements that match the tag name provided. This returns an array even if there is only a single match such as in this example where we look for the main tag.
Because we are only interested in the first element of the array we access it with the 0th index just as we did in Python.
document.getElementsByTagName("main")[0]
Arrays in Javascript behave just the same as they did in the Python course.
The elements begin at 0.
There is a built in length attribute to an array you can use to get an integer showing how many elements are in an array.
number_of_elements = my_array.length;
onmouseup
The onmouseup event occurs when the user releases the mouse button over an element. Add the following Javascript to either your HTML file or separate script file.
function mouseUp() {
document.getElementsByTagName("main")[0].style.backgroundColor = "#bc7887";
}
Then update your demo div to include this new event:
<div id="demo" onmouseout="mouseOut()" onmouseover="mouseOver()" onmousedown="mouseDown()" onmouseup="mouseUp()"> </div>
Save the files and reload the page and then click inside the square. The background colour of the main element should change to a dark blue when you click the mouse button down, but then change to a dark pink when you release it.
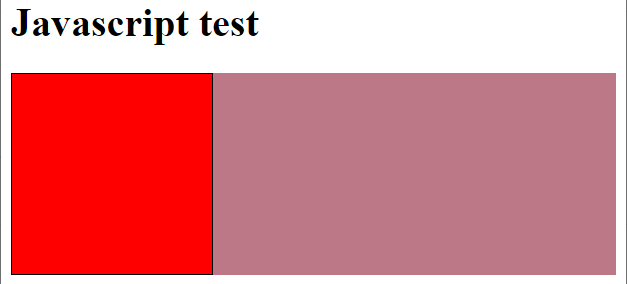
onclick
The onclick event occurs when the user clicks on an element such as a button or hyperlink. It is one event triggered after the mouse has been clicked down and then released. Add the following Javascript to either your HTML file or separate script file.
function mouseClick() {
var menu_matches = document.getElementsByClassName("menu1");
var counter;
for (counter = 0; counter< menu_matches.length; counter++) {
menu_matches[counter].style.backgroundColor = "red";
}
}
Then update your demo div to include this new event and a list underneath:
<div id="demo" onmouseout="mouseOut()" onmouseover="mouseOver()" onmousedown="mouseDown()"
onmouseup="mouseUp()" onclick="mouseClick()"> </div>
<ul>
<li class="list1">1</li>
<li>2</li>
<li class="list1">3</li>
<li>4</li>
</ul>
Save the files and reload the page and then click inside the square. The list elements that had the list1 class now have a background colour.
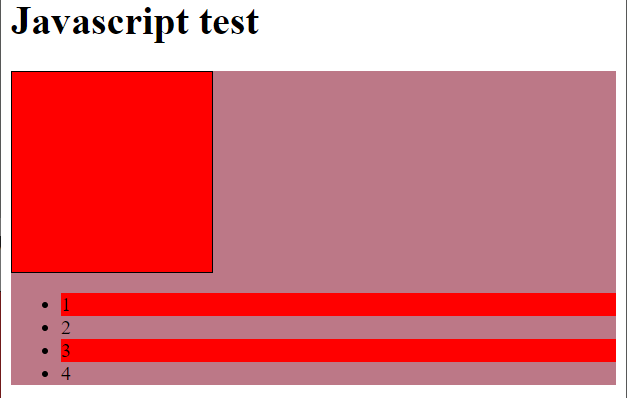
In Javascript you can assign a variable using the var keyword.
var counter;
The value can be set when the variable is assigned or at a later time.
A for loop in Javascript has 3 parameters in the brackets that control how many times the code will go through the loop.
for (counter = 0; counter< menu_matches.length; counter++) {
…
}
The first part sets an initial value to a variable.
counter = 0
The second part compares the variable against another variable or value each time around the loop to see if the loop should continue. This needs to return a Boolean true or false.
counter < menu_matches.length
The third part changes the value of the variable at the end of the current pass through the loop.
counter++
onmousemove
The onmousemove event occurs when the cursor is moving over an element such as an image or menu.
onmousemove happens whenever the mouse moves over the element whereas onmouseover only happens when the mouse enters the element or any child elements. Add the following to you Javascript:
var height_size = 50;
function mouseMove() {
height_size += 1;
document.getElementById("demo").style.height = height_size + "px";
}
And change your demo div code to be:
<div id="demo" onmouseout="mouseOut()" onmouseover="mouseOver()" onmousedown="mouseDown()"
onmouseup="mouseUp()" onclick="mouseClick()" onmousemove="mouseMove()"> </div>
Save your code and reload the page. Check what happens when you move the mouse around in the square. The square should start changing height as you move the mouse within it.
Like in Python you can concatenate two or more strings together with a + sign.
Unlike Python, Javascript is less strict and will automatically convert the integer into a string when it is concatenated with a string.
Printing
Browsers have a set of built-in functions that include the ability to print the screen. You can call that with the window.print() line.
<a href="#" onclick="window.print()">Print</a>
Final thoughts
Javascript is powerful. You can do a lot with Javascript.
You can validate forms to make sure that all fields have been filled out but no field has had too much data dumped into it which might identify a robot.
You can collapse and expand various elements on the page based on where the user is looking.
You can make feature rich interactive galleries that improve the experience for the user far beyond what is capable with just HTML and CSS.
But…
Javascript and any other client-side scripting is inherently unreliable.
You don’t know if a user, their browser, their anti-virus software, their company policies or anything else have disabled or limited client-side features. Because of this you cannot rely on client-side scripting to work.
Javascript should be used to improve the experience but the experience should not rely on it.